本文最后更新于 2025-04-12T09:49:25+00:00
前提条件
配置
在supabase上创建一个新项目
-
google cloud创建OAuth
google cloud创建一个新的项目,搜索OAuth找到OAuth Consent Screen,User Type选择external并创建。如果已有app可以edit the app重新编辑。
-
创建OAuth client
左侧栏Credentials创建一个客户端
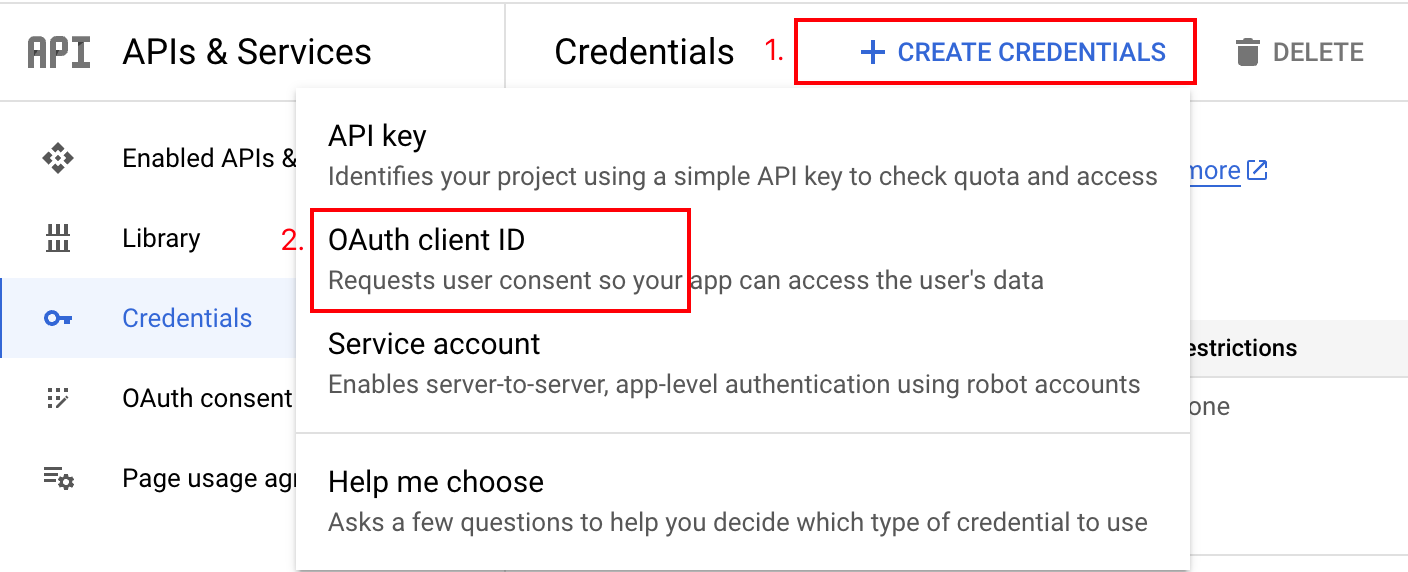
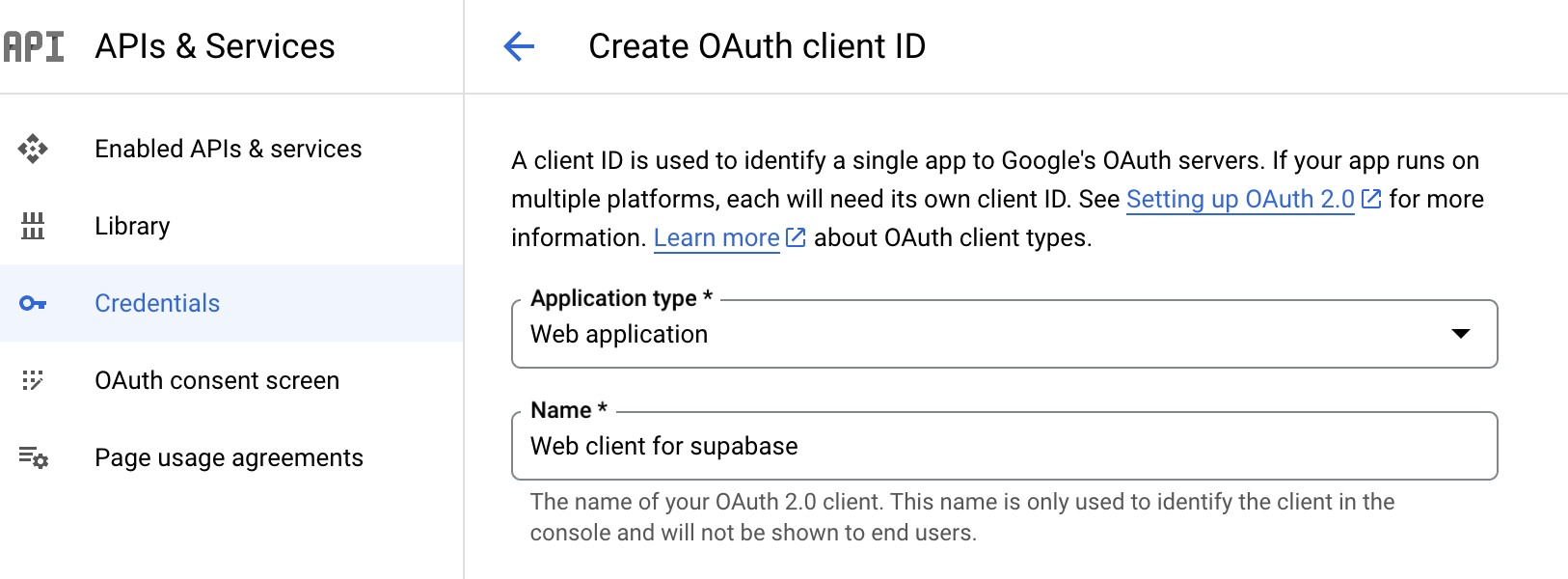
在Authorized redirect URIs添加上对应supabase项目的callback URL
https://<project-id>.supabase.co/auth/v1/callback
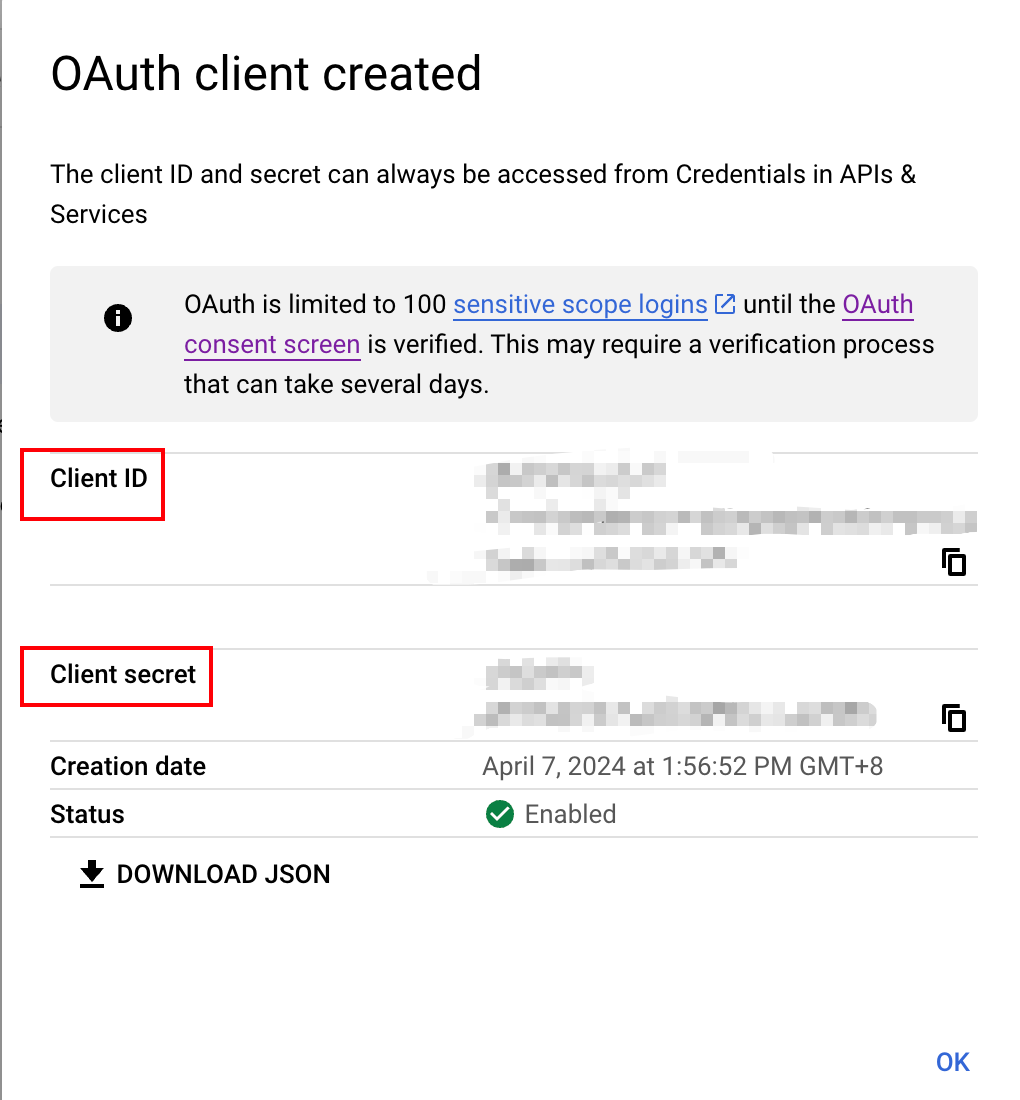
- supabase项目填写Google client id/secret
到supabase到dashboard点开项目找到Authentication-Providers -> Google
复制填好后点击save保存
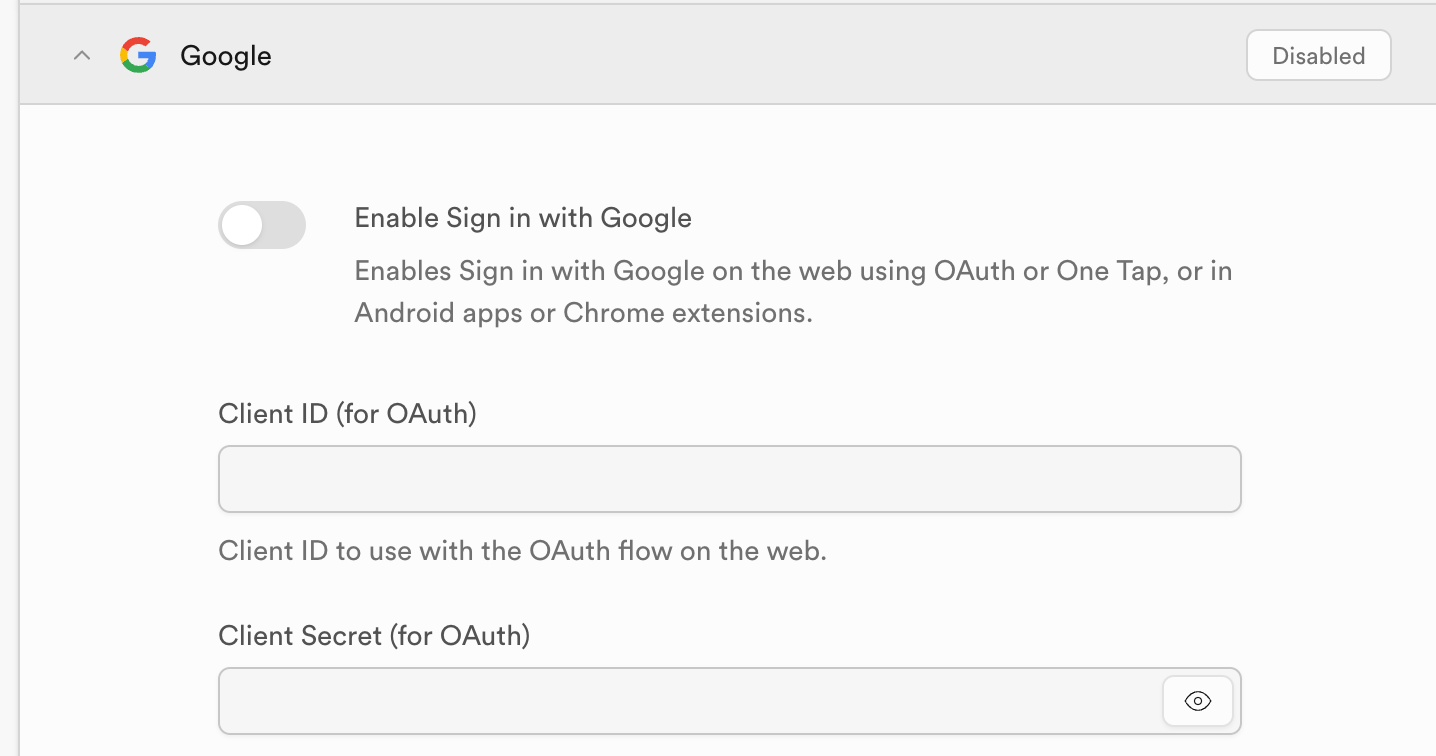
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
|
import { AuthChangeEvent, Session, createClient } from "@supabase/supabase-js"; import { getURL } from "next/dist/shared/lib/utils";
const supabaseUrl = process.env.NEXT_PUBLIC_SUPABASE_URL || ''; const supabaseAnonKey = process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY || '';
if (!supabaseUrl) throw new Error('Supabase URL not found.'); if (!supabaseAnonKey) throw new Error('Supabase Anon key not found.');
const supabase = createClient(supabaseUrl, supabaseAnonKey);
export function loginWithGoogle() { return supabase.auth.signInWithOAuth({ provider: 'google', options: { queryParams: { access_type: 'offline', prompt: 'consent', }, redirectTo: getURL() } }) }
export function logout() { return supabase.auth.signOut() }
export function onAuthStateChangedHelper(callback: ((event: AuthChangeEvent, session: Session | null) => void)) {
return supabase.auth.onAuthStateChange(callback) }
|
根据是否存在user,登录状态不同
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| import { loginWithGoogle, logout } from "../supabase/supabase"; import styles from './signIn.module.css' import { User } from "@supabase/auth-js"
interface SignInProps { user: User | null; }
export default function SignIn({ user }: SignInProps) { return ( <div> {user ? ( <button className={styles.signbtn} onClick={logout}>Sign Out</button> ) : ( <button className={styles.signbtn} onClick={loginWithGoogle}>Sign In</button> )} </div> )
}
|
用户变化用到onAuthStateChangedHelper
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| ...
const [user, setUser] = useState<User | null>(null);
useEffect(() => { const { data: authListener } = onAuthStateChangedHelper(async (event, session) => { console.log(`Supabase auth event: ${event}`); setUser(session?.user ?? null); }); return () => { authListener.subscription?.unsubscribe(); }; }, [])
...
|
参考链接
https://supabase.com/docs/guides/auth/social-login/auth-google?platform=web#configuration-web
https://www.youtube.com/watch?v=dE2vtnv83Fc&t=9s
https://supabase.com/docs/reference/javascript/auth-signout
https://ruanmartinelli.com/blog/supabase-authentication-react